AN ISO 29990 : 2010 & 9001 : 2015 CERTIFIED
DATA SCIENCE
Numpy :
-
NumPy is a fundamental Python library used for scientific and numerical computing.
-
Its name stands for Numerical Python, and it is the core library for working with arrays, which are like advanced lists that allow you to perform mathematical operations efficiently.
-
Multidimensional array object, and has functions and tools for working with these arrays.
-
pip install numpy
Import NumPy
Why Use NumPy?
-
Fast Calculations: It enables fast operations on large arrays and matrices because it is implemented in C and optimized for performance.
-
Multidimensional Arrays: You can create arrays of any dimension (1D, 2D, 3D, etc.), making it easier to work with data like tables (matrices) or even higher-dimensional data.
-
Mathematical Operations: It offers a range of operations like addition, subtraction, and multiplication that work element-wise on arrays.
-
Used in Data Science: NumPy is the base for many other libraries like Pandas, SciPy, and frameworks like TensorFlow.
Basic Concepts
1. ndarray (N-dimensional Array)
NumPy is an N-dimensional array type called ndarray.
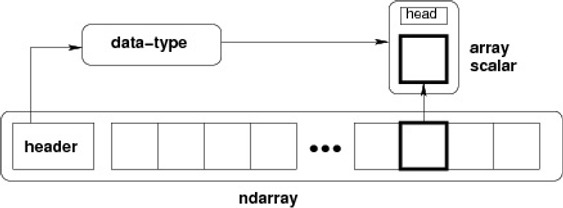
Example: Create a One-dimensional Array
import numpy
a = numpy.array([1, 2, 3, 4, 5])
print(a)
Output:
[1 2 3 4 5]
Example: Create a Multi-dimensional Array
import numpy as np
arr_2d = np.array([[1, 2, 3], [4, 5, 6]])
print(arr_2d)
Output:
[[1 2 3]
[4 5 6]]
2. Array Operations
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6]) # Element-wise addition
result = arr1 + arr2
print(result)
Output:
[5 7 9]
3. Shape and Size of an Array
arr = np.array([[1, 2, 3], [4, 5, 6]])
# Get shape (rows, columns)
print(arr.shape)
# Get the total number of elements
print(arr.size)
(2, 3) # 2 rows and 3 columns
6 # Total number of elements
Output:
4. Reshaping Arrays
arr = np.array([1, 2, 3, 4, 5, 6])
# Reshape to 2 rows and 3 columns
reshaped = arr.reshape(2, 3)
print(reshaped)
Output:
[[1 2 3]
[4 5 6]]